This program reverses a string entered by the user. For example if a user enters a string "reverse me" then on reversing the string will be "em esrever". We show you three different methods to reverse string the first one uses strrev library function of string.h header file and in second we make our own function to
reverse string using pointers,
reverse string using recursion and
Reverse words in string. If you are using first method then you must include string.h in your program.
#include <stdio.h>
#include <string.h>
int main()
{
char arr[100];
printf("Enter a string to reverse\n");
gets(arr);
strrev(arr);
printf("Reverse of entered string is \n%s\n",arr);
return 0;
}
Output of program:
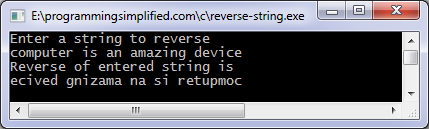
/* Second method */
C program to reverse a string using pointers
: Now we will invert string using pointers or without using library function strrev.
#include<stdio.h>
int string_length(char*);
void reverse(char*);
main()
{
char string[100];
printf("Enter a string\n");
gets(string);
reverse(string);
printf("Reverse of entered string is \"%s\".\n", string);
return 0;
}
void reverse(char *string)
{
int length, c;
char *begin, *end, temp;
length = string_length(string);
begin = string;
end = string;
for ( c = 0 ; c < ( length - 1 ) ; c++ )
end++;
for ( c = 0 ; c < length/2 ; c++ )
{
temp = *end;
*end = *begin;
*begin = temp;
begin++;
end--;
}
}
int string_length(char *pointer)
{
int c = 0;
while( *(pointer+c) != '\0' )
c++;
return c;
}
C program to reverse a string using recursion
#include <stdio.h>
#include <string.h>
void reverse(char*, int, int);
int main()
{
char a[100];
gets(a);
reverse(a, 0, strlen(a)-1);
printf("%s\n",a);
return 0;
}
void reverse(char *x, int begin, int end)
{
char c;
if (begin >= end)
return;
c = *(x+begin);
*(x+begin) = *(x+end);
*(x+end) = c;
reverse(x, ++begin, --end);
}
In recursion method we swap characters at the begin and at the end and then move towards the middle of the string. This method is inefficient due to repeated function calls but useful in practicing recursion.
No comments:
Post a Comment
Tell Us What You've Got...